In ASP.NET, you can
access services at any stage of your HTTP pipeline through dependency
injection. By default, it provides Constructor injection, but it can easily be
replaced by any other container of your choice. Before moving ahead, one
point is very important to understand and that is lifetime of
services.
ASP.NET Core allows
you to configure four different lifetimes for your services.
- Transient - Created on every request
- Scoped - Created once per request
- Singleton - Created first time when they are requested
- Instance - Created in ConfigureServices method and behaves like a Singleton
To understand the
service consumption in easier way, we will create a sample application going
step-by-step. My example service will provide GUID to respective controller.
So, let's start quickly.
Create a new project - Create a new project using ASP.NET Web Application
template as shown below:
Add Service Interface - Create an interface named IGUIDService under Services folder as shown below:
Add Service Implementation - Create a class named GUIDServiceunder Services folder and provide the implementation of IGUIDService interface as shown below:
Add a Controller - Next task is to add a Controller namedGUIDController by right clicking on Controllers folder as shown below: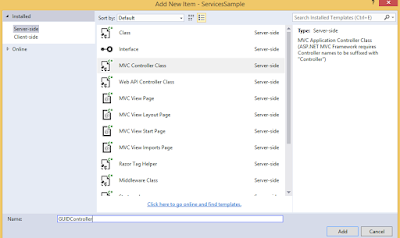
Add a View - Before adding a View, create a folder named under Views folder. Now add a View by right clicking on GUID folder as shown:
Update the code inside View as shown below:
Use Service in Controller - Now instantiate the service and set the data for View as shown below:
Register Service - Last step is to register the service in the ConfigureServices method of Startup class as shown below:
Everything is set. Now run your application and you would be able to see GUID on browser as:
Add Service Interface - Create an interface named IGUIDService under Services folder as shown below:
Add Service Implementation - Create a class named GUIDServiceunder Services folder and provide the implementation of IGUIDService interface as shown below:
Add a Controller - Next task is to add a Controller namedGUIDController by right clicking on Controllers folder as shown below:
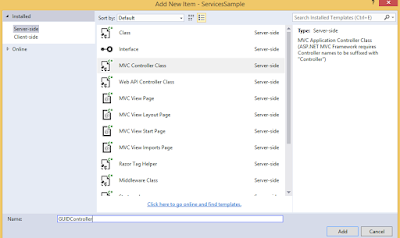
Add a View - Before adding a View, create a folder named under Views folder. Now add a View by right clicking on GUID folder as shown:
Update the code inside View as shown below:
Use Service in Controller - Now instantiate the service and set the data for View as shown below:
Register Service - Last step is to register the service in the ConfigureServices method of Startup class as shown below:
Everything is set. Now run your application and you would be able to see GUID on browser as:
Comments
Post a Comment